Vue Js Convert Json Object to Base64:In Vue.js, you can convert a JSON object to a Base64 string using the btoa() function. To do this, first convert the JSON object to a string using JSON.stringify(), and then use the btoa() function to encode the string to a Base64 string. This method is useful when you need to send or store the JSON data in a format that can be easily transmitted or saved. The btoa() function is built-in and works in most modern browsers,
How can you Vue Js convert a JSON object to a Base64 encoded string?
The code you provided is using the btoa()
function to convert a JSON object to a Base64-encoded string. Here’s a breakdown of what’s happening:
- The
convertToBase64()
method is being called when the component is mounted (mounted()
). - The
for
loop iterates over each object inmyObjects
. JSON.stringify()
is used to convert each object to a JSON string.btoa()
is used to convert the JSON string to a Base64-encoded string.- The Base64-encoded string is pushed to an array called
base64Strings
.
Vue Js Convert Json Object to Base64 Example
<div id="app">
<p>JSON Objects:</p>
<pre>{{ myObjects }}</pre>
<p>Base64 Strings:</p>
<pre>{{ base64Strings }}</pre>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
myObjects: [
{
name: "Alice",
age: 28,
email: "alice@example.com"
},
{
name: "Bob",
age: 35,
contacts: [
{
name: "Alice",
email: "alice@example.com"
},
{
name: "Charlie",
email: "charlie@example.com"
}
],
address: {
street: "123 Main St",
city: "Anytown",
state: "CA",
zip: "12345"
}
}
],
base64Strings: []
};
},
methods: {
convertToBase64() {
for (let obj of this.myObjects) {
const jsonString = JSON.stringify(obj);
this.base64Strings.push(btoa(jsonString));
}
}
},
mounted() {
this.convertToBase64();
}
})
</script>
Output of Vue Js Convert Json Object to Base64
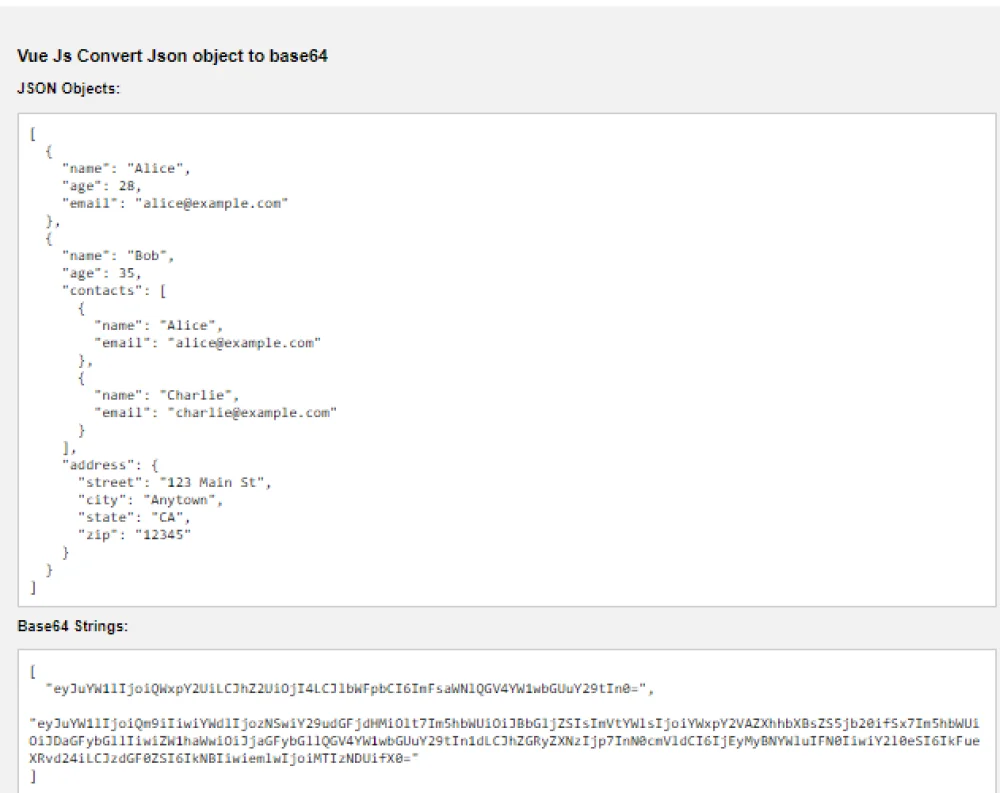